Writing High-Performance Embedded C Code: Tips, Tricks, and Real-World Examples
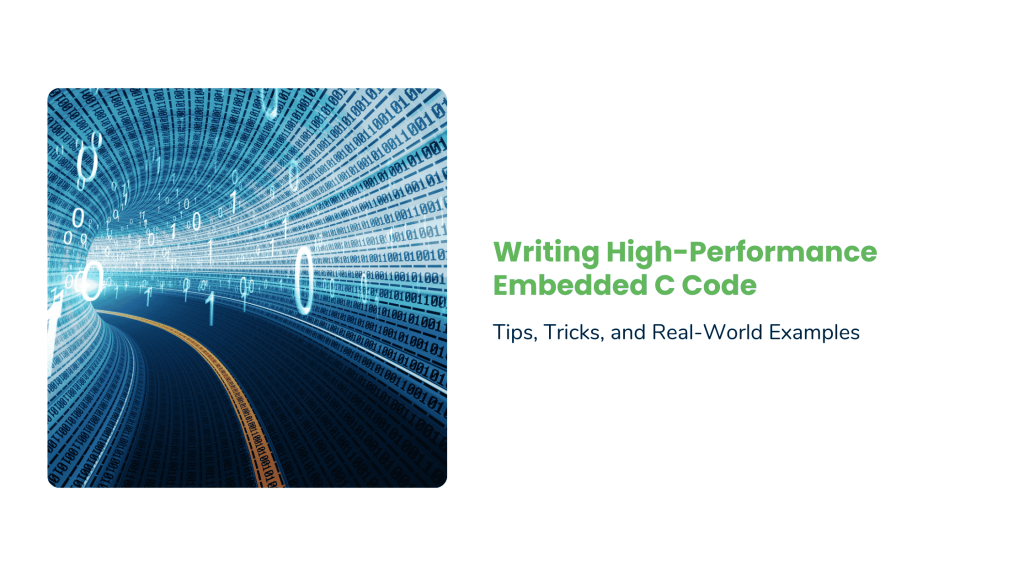
Introduction
Embedded systems often operate under strict performance and resource constraints, making it essential to write efficient and optimized C code. In this blog, we’ll explore practical techniques to improve execution speed, memory usage, and power efficiency with hands-on examples.
-
Optimize Memory Usage
Efficient memory handling is critical in embedded systems where RAM is limited.
Use Fixed-Size Data Types:
Unsigned Char (unsigned char)
Definition:
unsigned char is an 8-bit data type (1 byte) that holds values from 0 to 255.
Usage in Embedded Systems:
- Memory Efficiency: Used when only small values are needed, reducing RAM usage.
- Portability: Ensures consistent behavior across platforms where char may be signed or unsigned by default.
- Hardware Registers & Buffers: Often used to interface with hardware registers or handle communication buffers.
Example:
unsigned char sensorData = 200; // Example sensor reading
Understanding uint32_t
Definition:
uint32_t is a 32-bit (4-byte) unsigned integer that stores values from 0 to 4,294,967,295.
Why Use uint32_t?
- Fixed-Size Guarantee: Ensures a consistent 32-bit representation across different compilers.
- Performance Optimization: Helps optimize memory usage and performance on 32-bit microcontrollers.
- Interfacing with Hardware Registers: Many microcontroller peripherals use 32-bit registers.
Example:
uint32_t adcReading = 123456789; // Example ADC value
Understanding Bitfields in Embedded C
Definition:
Bitfields allow efficient memory usage by defining a structure where individual bits or groups of bits represent values.
Why Use Bitfields?
- Memory Efficiency: Saves memory by packing multiple fields into a single byte or word.
- Register-Level Programming: Used to map microcontroller hardware registers efficiently.
- Improves Code Readability: Provides a structured way to represent bitwise data
Avoid Dynamic Memory Allocation:
Heap allocation (malloc/free) can lead to fragmentation and unpredictable behavior.
-
Minimize CPU Cycles with Efficient Loops
Unoptimized loops can consume unnecessary CPU cycles.
Use Loop Unrolling for Small Iterations:
This reduces loop overhead and improves execution speed.
-
Leverage Compiler Optimizations
Modern compilers offer powerful optimization flags to enhance performance.
Enable Compiler Optimizations (GCC Example):
-O2 enables aggressive optimizations without significantly increasing code size.
-
Use Volatile for Interrupts & Shared Variables
When dealing with hardware registers and interrupts, use volatile to prevent unwanted compiler optimizations.
-
Reduce Function Call Overhead
Function calls introduce stack operations that slow execution. For frequently used small functions, use inline.
This eliminates the function call overhead, inlining the code directly where used.
-
Utilize DMA for Peripheral Communication
Using Direct Memory Access (DMA) offloads CPU workload for data transfers.
Example: Configuring DMA for UART on STM32:
This frees up CPU cycles while the transfer occurs in the background.
Conclusion
Writing high-performance embedded C code requires careful attention to memory, CPU cycles, and hardware-specific optimizations. Developers can create efficient, fast, and power-optimized embedded applications by applying these techniques.